This website uses cookies so that we can provide you with the best user experience possible. Cookie information is stored in your browser and performs functions such as recognising you when you return to our website and helping our team to understand which sections of the website you find most interesting and useful.
OPC UA
Client & Server
in C# / VB.NET
quick and easy.
Writing an OPC UA Client like a breeze:
var client = new OpcClient("opc.tcp://localhost:4840");
client.Connect();
var temperature = client.ReadNode("ns=2;s=Temperature");
Console.WriteLine("Temperature is {0} °C", temperature);
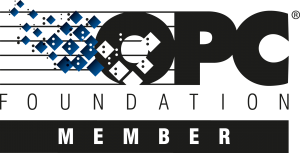
Your Benefits
Simple and fast development
Write your OPC UA application with a few lines of code and save time and money. Get a minimal Time-to-Market.
You can focus on your project while our SDK takes care about complexity of the OPC UA communication standard.
Industrial grade reliability
After more than 25 years of experience in the field of industrial communication we are aware of the challenges and problems that your applications have to conquer in every day life of industrial automation.
To ensure a flawless 24/7 operation we pay severe attention to the stability and compatibility of our software.
API design according to Microsoft standards
During the development of our SDKs we are following the Microsoft standards for framework design. This enables you to get started effortlessly with developing your application while you benefit from a modern API and in-depth debugging capabilities.
Royalty-free Licenses
Our licensing model allows you to develop and distribute as many applications as you like based on the OPC UA SDK – without any additional license fee.
Qualified Support
We are very interested in learning from the problems and applications of our customers. This allows us to continuously adapt and improve our products.
For this reason, we provide qualified support directly from our developers.
Many code examples
To get you started quickly, we provide a steadily growing number of code examples via our public GitHub repository.
Besides basic standard use cases these examples comprise implementations of real-world scenarios that are often requested by our customers.
Periodical Updates
Our OPC UA SDK is under constant development. We are always keen on hearing about your use cases in order to be able to improve and extend our framework. In this way we can provide you with updates containing bug fixes and new features on a regular basis.
Features
Security Policies
None • Sign • SignAndEncrypt
Security Algorythm
Auto • Custom • None • Basic128Ras15 • Basic256 • Basic256Sha256 • Https
Configuration
In Code • XML-File • dynamic
Nodemanager
Browse • Read • Write • Add • Delete
Easy Node Access
Access Node by Browse Name • NodeId
Data Access
DA • HDA • Alarm • Conditions • Events • Methods • Subscription • Custom Datatype • File
Structured Data Types
To help you with your individual use case, the SDK provides several ways to use Custom Data Types in your application.
Name-Value pairs
The access to properties of a structured data type is analogous to a dictionary by means of the name of the property.
.NET dynamic
The structured data type is used as .NET dynamic and can therefore be used analogously to a .NET type.
.NET Types
Structured data types can also be defined as .NET Types and registered in the SDK. This allows you to use it in a type-safe way.
The .NET code can be created automatically via the supplied OPC UA Tool and integrated directly into your project.
Are you looking for a feature?
Supported Platforms
Our OPC UA SDK is compliant to
.NET Standard 2.0
Thus, you can develop applications for the following target frameworks:
.NET Framework
.NET Core
Mono
Xamarin
Universal Windows Platform
Unity
iOS
Android
Linux
Windows
macOS
Code Examples
C# OPC UA Client
This OPC UA client establishes a connection to a local server, reads periodically the value of a node and prints it to the console.
using System;
using System.Threading;
using Opc.UaFx.Client;
public class Program
{
public static void Main()
{
using (var client = new OpcClient("opc.tcp://localhost:4840")) {
client.Connect();
while (true) {
var temperature = client.ReadNode("ns=2;s=Temperature");
Console.WriteLine("Current Temperature is {0} °C", temperature);
Thread.Sleep(1000);
}
}
}
}
using System.Threading; using Opc.UaFx; using Opc.UaFx.Server; class Program { public static void Main() { var temperatureNode = new OpcDataVariableNode<double>("Temperature", 100.0); using (var server = new OpcServer("opc.tcp://localhost:4840/", temperatureNode)) { server.Start(); while (true) { if (temperatureNode.Value == 110) temperatureNode.Value = 100; else temperatureNode.Value++; temperatureNode.ApplyChanges(server.SystemContext); Thread.Sleep(1000); } } } }
C# OPC UA Server
This OPC UA server provides a local node whose value is changed periodically.
Do you want to evaluate the SDK?
License model
Single Developer License
- Applies to a single person who is registered by name as a developer
- One single developer is allowed to use the SDK
- Any product / application developed with the SDK may be deployed in unlimited number to your customers and may be used by them (royalty free).
- Unlimited life time of the license
- Perfectly suitable for developing standard products
Branch License
- Applies to one branch (= a postal address), registered for developing
- Unlimited number of developers is allowed to use the SDK
- Any product / application developed with the SDK may be deployed in unlimited number to your customers and may be used by them (royalty free)
- Unlimited life time of the license
- Perfectly suitable for developing standard products
Downloads
Client
With its minimal deployment size the pure client SDK can even be deployed on embedded devices.
Server
The Server SDK raises your data sources to the industrial OPC UA Standard.
Client + Server Bundle
Client and server functionality combined in one package, ready to develop extensive OPC UA applications.
Using the SDK as NuGet package:
For the Client SDK:
PM> Install-Package Opc.UaFx.Client
For the Server or Client+Server Bundle SDK:
PM> Install-Package Opc.UaFx.Advanced
For the Client SDK:
> dotnet add package Opc.UaFx.Client
For the Server or Client+Server Bundle SDK:
> dotnet add package Opc.UaFx.Advanced
For the Client SDK:
<PackageReference Include="Opc.UaFx.Client" />
For the Server or Client+Server Bundle SDK:
<PackageReference Include="Opc.UaFx.Advanced" />
Für Projekte, die PackageReference unterstützen, kopieren Sie folgendes XML-Element in die Projekt-Datei, um das Paket zu referenzieren.
Prices
Client
Single Developer License-
for one single developer
-
incl. 12 months Top Level Support
-
unlimited number of applications
-
unlimited license lifetime
-
royalty free
-
Item no.:9780.net-Client-D
-
With additional
OPC Classic Option1980,- € + VATItem no.:
9782.net-Client-DSupport renewal:
297,- € + VAT
148,50 € + VAT
Server
Single Developer License-
for one single developer
-
incl. 12 months Top Level Support
-
unlimited number of applications
-
unlimited license lifetime
-
royalty free
-
Item-no.:9780.net-Server-D
326,25 € + VAT
Client / Server Bundle
Single Developer License-
for one single developer
-
incl. 12 months Top Level Support
-
unlimited count of applications
-
unlimited license lifetime
-
royalty free
-
Item-no.:9780.net-CLSV-D
-
With additional
OPC Classic Option3590,- € + VATItem no.:
9782.net-CLSV-DSupport renewal:
538,50 € + VAT
390,00 € + VAT
Client
Branch License-
unlimited number of developers at your branch
-
incl. 12 months Top Level Support
-
unlimited count of applications
-
unlimited license lifetime
-
royalty free
-
Item no.:
9780.net-Client -
With additional
OPC Classic Option4000,- € + VATItem no.:
9782.net-ClientSupport renewal:
600,- € + VAT
300,00 € + VAT
Server
Branch License-
unlimited number of developers at your branch
-
incl. 12 months Top Level Support
-
unlimited count of applications
-
unlimited license lifetime
-
royalty free
-
Item no.:
9780.net-Server
652,50 € + VAT
Client / Server Bundle
Branch License-
unlimited number of developers at your branch
-
incl. 12 months Top Level Support
-
unlimited count of applications
-
unlimited license lifetime
-
royalty free
-
Item no.:
9780.net-CLSV -
With additional
OPC Classic Option7200,- € + VATItem no.:
9782.net-CLSVSupport renewal:
1080,- € + VAT
780,00 € + VAT
Contact
OPC UA
Client & Server
in C# / VB.NET
quick and easy.
Submit your request.
You’ll automatically get the info package:
- Prices and order Information
- The Evaluation Package – You can get started immediately
- Manual
Traeger Industry Components GmbH
Söllnerstrasse 9
92637 Weiden
Germany
info@traeger.de
+49 961 48230-0
By submitting this form you accept our data protection regulations.